Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Tags
- python
- tableau
- 데이터 핸들링
- 컨브넷
- XGBoost
- Growth hacking
- 인프런
- splitlines
- 그로스 해킹
- ARIMA
- 분석 패널
- WITH CUBE
- 캐글 신용카드 사기 검출
- WITH ROLLUP
- 그로스 마케팅
- DENSE_RANK()
- 데이터 증식
- lightgbm
- 부트 스트래핑
- pmdarima
- 그룹 연산
- 리프 중심 트리 분할
- ImageDateGenerator
- 로그 변환
- 스태킹 앙상블
- 마케팅 보다는 취준 강연 같다(?)
- 3기가 마지막이라니..!
- 데이터 정합성
- sql
- 캐글 산탄데르 고객 만족 예측
Archives
- Today
- Total
LITTLE BY LITTLE
[3,실습] 케라스 창시자에게 배우는 딥러닝 - 신경망 시작하기 (분류/회귀 문제 풀어보기 본문
3. 신경망 시작하기¶
In [286]:
pip install keras
Requirement already satisfied: keras in c:\users\lh2275\anaconda3\lib\site-packages (2.9.0)
Note: you may need to restart the kernel to use updated packages.
3.1.1 층 : 딥러닝의 구성 단위¶
In [287]:
from keras import layers
layer = layers.Dense( 32, input_shape = (784, ))
# 32개 유닛으로 밀집된 층 -> 차원 크기가 32로 변환된 텐서를 출력할 것
# 1번째 차원이 784인 2D텐서만 입력으로 받는 층, 배치차원인 0번째 축은 지정하지 않음 (어떤 크기의 입력도 받을 수 있음)
# layers.Dense 입출력 연결 기능
In [288]:
from keras import models
from keras import layers
model = models.Sequential()
model.add(layers.Dense(32,input_shape=(784,)))
model.add(layers.Dense(10))
# Sequential 모델은 계층을 선형으로 쌓은 것
# add 연산자로 쉽게 추가 가능
3.2.2 모델 : 케라스를 사용한 개발 - 빠르게 둘러보기¶
In [289]:
# Sequential 클래스를 사용하여 정의한 2채의 층으로된 모델
from keras import models
from keras import layers
model = models.Sequential()
model.add(layers.Dense(32,activation='relu', input_shape=(784,))) #1번째 층에 입력 데이터 크기가 전달됨
model.add(layers.Dense(10,activation='softmax'))
# 같은 모델을 Sequential이 아닌 함수형 API로 모델 정의
input_tensor = layers.Input(shape=(784,)) # Input에 I 대문자임 주의
x = layers.Dense(32,activation='relu')(input_tensor) # 모델이 처리할 데이터 텐서를 먼저 만든다.
output_tensor = layers.Dense(10,activation='softmax')(x)
model = models.Model(inputs=input_tensor, outputs=output_tensor) # 함수처럼 텐서에 층을 적용
# Model에 M 대문자임 주의 ( 다 대문자임 위에 .Dense도 대문자 ..)
In [290]:
# 손실함수 사용 - 컴파일할 때.
from keras import optimizers
model.compile(optimizer = optimizers.RMSprop(learning_rate=0.001),loss='mse',metrics=['accuracy'])
# model.compile()
# 원래 lr=0.001로 줄여썼지만, deprecated되었다고 learning_rate로 쓰라는 warning이 나옴
In [291]:
# 모델을 Sequential 혹은 함수형 API로 정의한 후 , 컴파일하고, 마지막으로 FIT()으로 학습
# 예시 model.fit(input_tensor, target_tensor, batch_size = 128, epochs=10)
이진 분류 - 영화 리뷰 분류 예제¶
In [292]:
from keras.datasets import imdb
(train_data, train_labels), (test_data, test_labels) = imdb.load_data(num_words=10000)
#이진 분류라서 여기서 label은 긍정리뷰 = 1, 부정리뷰 = 0
# num_words를 10,000개로 제한하여 단어 인덱스가 9,999를 넘지 않음
In [293]:
train_data[0]
# 전처리가 되어있는 데이터라 단어 시퀀스가 숫자 시퀀스로 변환된 형태로 나온다.
# (각 숫자는 고유한 단어를 나타냄)
Out[293]:
[1,
14,
22,
16,
43,
530,
973,
1622,
...
5345,
19,
178,
32]
In [294]:
train_labels[0]
Out[294]:
1
In [295]:
max([max(sequence) for sequence in train_data])
Out[295]:
9999
In [296]:
# 숫자 시퀀스로 전처리된 리뷰 데이터 하나를 다시 단어 시퀀스로 바꿔보자.(원래 형태 확인)
word_index = imdb.get_word_index()
reverse_word_index = dict([(value,key) for (key,value) in word_index.items()])
decoded_review = ' '.join([reverse_word_index.get(i-3,'?') for i in train_data[0]])
print(decoded_review)
# word_index -> word_index는 단어와 정수 인덱스를 매핑한 딕셔너리
# reverses_word_index -> 정수 인덱스와 단어를 매핑하도록 뒤집기
# decoded_review -> 리뷰 디코딩하기. i-3을 한 이유는 0,1,2가 '패딩','문서시작','사전에 없음'을 위한 인덱스임
# get() 은 i-3에 해당하는 key값이 존재할 경우 그 값을, 없을 경우 지정한 문자 '?'를 반환한다.
? this film was just brilliant casting location scenery story direction everyone's really suited the part they played and you could just imagine being there robert ? is an amazing actor and now the same being director ? father came from the same scottish island as myself so i loved the fact there was a real connection with this film the witty remarks throughout the film were great it was just brilliant so much that i bought the film as soon as it was released for ? and would recommend it to everyone to watch and the fly fishing was amazing really cried at the end it was so sad and you know what they say if you cry at a film it must have been good and this definitely was also ? to the two little boy's that played the ? of norman and paul they were just brilliant children are often left out of the ? list i think because the stars that play them all grown up are such a big profile for the whole film but these children are amazing and should be praised for what they have done don't you think the whole story was so lovely because it was true and was someone's life after all that was shared with us all
In [297]:
# 데이터를 불러온 후, 신경망에 숫자 리스트를 주입할 수 없기 때문에, 리스트->텐서 작업해주기
# 정수 시퀀스를 이진 행렬로 인코딩하기. (원-핫 인코딩으로 0과 1의벡터로 변환하기)
import numpy as np
def vectorize_sequences(sequences, dimension=10000):
results = np.zeros((len(sequences),dimension))
for i, sequence in enumerate(sequences):
results[i,sequence] = 1.
return results
x_train = vectorize_sequences(train_data)
x_test = vectorize_sequences(test_data)
x_train[0]
# 벡터화 해주는 함수 생성
# results = 크기가 (len(sequences), dimension ) 이고, 모든 원소가 0인 행렬
# 앞서 num_words를 10,000개로 제한했으니 dimension을 10,000으로 똑같이 지정
# enumerate는 원소 뿐만 아니라 인덱스에도 접근하기 위해서 사용 -> (인덱스,원소) 형식으로 출력
# 앞서 np.zeros해준 results에 results[i,sequence] = 1 해주기
Out[297]:
array([0., 1., 1., ..., 0., 0., 0.])
In [298]:
# 레이블은 단순히 astype()으로 벡터로 바꿀 수 있다.
y_train = np.asarray(train_labels).astype('float32')
y_test = np.asarray(test_labels).astype('float32')
신경망 모델 만들기¶
- Dense 층을 쌓을 때 사용하는 일반적인 원리
- 중간에 있는 은닉 층은 활성화 함수 relu (*relu함수는 음수를 0으로 만듦) 사용
- 마지막 층은 확률(0과 1사이의 점수)을 출력하기 위해 시그모이드 활성화 함수 사용
- 위의 일반적인 원리를 따르는 신경망을 만들자.
In [299]:
# 1) 모델 정의하기
from keras import models
from keras import layers
model = models.Sequential()
model.add(layers.Dense(16,activation='relu', input_shape=(10000,)))
model.add(layers.Dense(16, activation='relu'))
model.add(layers.Dense(1, activation='sigmoid'))
In [300]:
# 2) 손실함수와 옵티마이저 선택
# 이진분류 문제고, 출력이 확률일 때에는 binary_crosscentropy 손실이 적합
# 3) 선택한 손실함수와 옵티마이저로 컴파일하기
model.compile(optimizer='rmsprop', loss='binary_crossentropy', metrics=['accuracy'])
In [301]:
# 만약 옵티마이저 매개변수를 바꾸고 싶은 경우
from keras import optimizers
model.compile(optimizer=optimizers.RMSprop(learning_rate=0.001), loss = 'binary_crossentropy',
metrics=['accuracy'])
In [302]:
# 만약 옵티마이저에 측정 함수를 전달해야할 경우 - 손실,측정을 함수 객체로 지정하기
from keras import losses
from keras import metrics
model.compile(optimizer=optimizers.RMSprop(learning_rate=0.001),
loss=losses.binary_crossentropy, metrics=[metrics.binary_accuracy])
- 활성화 함수(=비선형성)를 사용하는 이유는 가설공간을 확장하기 위함, 그 중 relu함수가 많이쓰인다.
훈련 검증¶
앞서 컴파일까지 완료하였다. 훈련 데이터에서 샘플을 떼서 검증세트로 만드는 작업을 해야한다.
In [303]:
# 훈련 중 모델의 정확도를 측정하기위해 '검증 세트'만들기
x_val = x_train[:10000]
partial_x_train = x_train[10000:]
y_val = y_train[:10000]
partial_y_train = y_train[10000:]
In [304]:
model.compile(optimizer='rmsprop', loss='binary_crossentropy', metrics=['acc'])
In [307]:
# 모델 훈련
history = model.fit(partial_x_train, partial_y_train, epochs=20, batch_size=512,
validation_data = (x_val, y_val),verbose=0)
# 훈련시킨 결과를 history에 저장
In [308]:
# history - 훈련하는 동안의 모든 데이터(손실,정확도..)를 담고있는 딕셔너리
history_dict = history.history
history_dict.keys()
Out[308]:
dict_keys(['loss', 'acc', 'val_loss', 'val_acc'])
In [309]:
# 훈련, 검증 데이터 손실 그래프 그려보기 (최적의 epoch 횟수를 찾기위함)
import matplotlib.pyplot as plt
history_dict = history.history
loss = history_dict['loss']
val_loss = history_dict['val_loss']
epochs = range(1, len(loss) + 1)
plt.plot(epochs, loss, 'bo', label='Training loss')
plt.plot(epochs, val_loss, 'b', label='Validation loss')
plt.xlabel('Epochs')
plt.ylabel('Loss')
plt.legend()
plt.show()
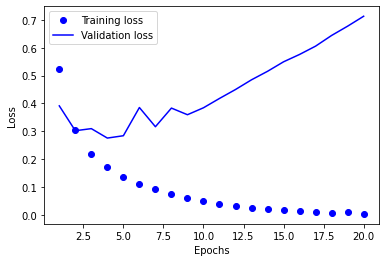
In [310]:
# 훈련,검정 데이터 정확도 그리기
plt.clf() # 그래프 초기화
acc = history_dict['acc']
val_acc = history_dict['val_acc']
plt.plot(epochs, acc, 'bo', label='Training acc')
plt.plot(epochs, val_acc, 'b', label='Validation acc')
plt.title('Training and Validation accuracy')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
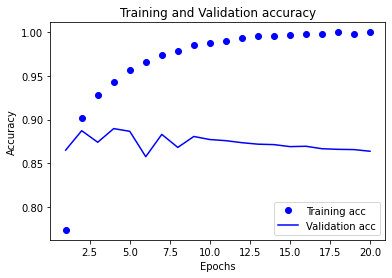
In [311]:
# training loss를 보면, epoch=4 이후에 과대적합이 발생 -> epoch=4로 설정하고 다시 훈련
model=models.Sequential()
model.add(layers.Dense(16,activation='relu',input_shape=(10000,)))
model.add(layers.Dense(16,activation='relu'))
model.add(layers.Dense(1,activation='sigmoid'))
model.compile(optimizer='rmsprop', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, epochs=4, batch_size=512)
results= model.evaluate(x_test, y_test)
# 최종 결과 (epoch=4로 훈련시 loss , acc값 출력)
results
Epoch 1/4
49/49 [==============================] - 2s 29ms/step - loss: 0.4734 - accuracy: 0.8110
Epoch 2/4
49/49 [==============================] - 1s 28ms/step - loss: 0.2681 - accuracy: 0.9073
Epoch 3/4
49/49 [==============================] - 1s 29ms/step - loss: 0.2028 - accuracy: 0.9285
Epoch 4/4
49/49 [==============================] - 1s 29ms/step - loss: 0.1699 - accuracy: 0.9401
782/782 [==============================] - 2s 2ms/step - loss: 0.2931 - accuracy: 0.8838
Out[311]:
[0.2931343913078308, 0.883840024471283]
모델 훈련 후, predict() 메소드로 어떤 리뷰가 긍정적일지 예측할 수 있다.
In [312]:
model.predict(x_test)
782/782 [==============================] - 2s 3ms/step
Out[312]:
array([[0.19381014],
[0.99983853],
[0.788698 ],
...,
[0.14890665],
[0.05754507],
[0.6090945 ]], dtype=float32)
다중 분류 - 뉴스 기사 분류 예제¶
In [313]:
import keras
from keras.datasets import reuters
(train_data, train_labels), (test_data, test_labels) = reuters.load_data(num_words=10000)
In [314]:
print('train데이터 개수:',len(train_data))
print('test데이터 개수:',len(test_data))
print(train_data[10])
train데이터 개수: 8982
test데이터 개수: 2246
[1, 245, 273, 207, 156, 53, 74, 160, 26, 14, 46, 296, 26, 39, 74, 2979, 3554, 14, 46, 4689, 4329, 86, 61, 3499, 4795, 14, 61, 451, 4329, 17, 12]
In [315]:
# 텍스트로 디코딩해보자(전처리 전 데이터가 어떻게 생겼는지 확인)
word_index = reuters.get_word_index()
reverse_word_index = dict([(value,key) for (key,value) in word_index.items()])
decoded_newswire = ' '.join([reverse_word_index.get(i-3,'?') for i in train_data[0]])
print(decoded_newswire)
? ? ? said as a result of its december acquisition of space co it expects earnings per share in 1987 of 1 15 to 1 30 dlrs per share up from 70 cts in 1986 the company said pretax net should rise to nine to 10 mln dlrs from six mln dlrs in 1986 and rental operation revenues to 19 to 22 mln dlrs from 12 5 mln dlrs it said cash flow per share this year should be 2 50 to three dlrs reuter 3
In [316]:
train_labels[10]
# 레이블은 토픽의 인덱스로, 0과 45사이 정수임
Out[316]:
3
In [317]:
# 데이터를 인코딩하자. (벡터로, 원-핫 인코딩 이용)
import numpy as np
def vectorize_sequences(sequences,dimension=10000):
results = np.zeros((len(sequences),dimension))
for i, sequence in enumerate(sequences):
results[i,sequence] = 1.
return results
x_train = vectorize_sequences(train_data)
x_test = vectorize_sequences(test_data)
In [318]:
# 레이블을 벡터로 바꾸자. to_categorical() 이용, 이진 분류에서는 astype() 이용했었음
from keras.utils.np_utils import to_categorical
one_hot_train_labels = to_categorical(train_labels)
one_hot_test_labels = to_categorical(test_labels)
In [319]:
# 모델 정의하기 (정보 병목 주의, 너무 작은 차원 쓰지 X -> 46차원으로)
from keras import models
from keras import layers
model = models.Sequential()
model.add(layers.Dense(64, activation='relu', input_shape=(10000,)))
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(46, activation='softmax')) #softmax 활성화함수는 확률분포를 출력해줌
어떤 옵티마이저, 손실함수 사용할지 결정 - 두 확률 분포 사이의 거리를 측정하는 categorical_crossentropy 사용
In [320]:
# 모델 컴파일하기
model.compile(optimizer='rmsprop', loss='categorical_crossentropy',metrics=['acc'])
In [321]:
# 훈련 검증
# 검증 세트 준비 - 훈련 데이터 8982개 중 1000개를 검증 데이터로 사용
x_val = x_train[:1000]
partial_x_train = x_train[1000:]
y_val = one_hot_train_labels[:1000]
partial_y_train = one_hot_train_labels[1000:]
In [323]:
# 모델 훈련하기(epochs=20)
history = model.fit(partial_x_train, partial_y_train, epochs=20, batch_size=512,
validation_data=(x_val,y_val),verbose=0)
In [324]:
# 훈련과 검증 손실 그래프 그리기
import matplotlib.pyplot as plt
loss = history.history['loss']
val_loss = history.history['val_loss']
epochs = range(1, len(loss) +1)
plt.plot(epochs, loss, 'bo', label='Training loss')
plt.plot(epochs, val_loss, 'b', label='Validation loss')
plt.title('Training and Validation loss')
plt.xlabel('Epochs')
plt.ylabel('Loss')
plt.legend()
plt.show()

In [325]:
history.history.keys()
Out[325]:
dict_keys(['loss', 'acc', 'val_loss', 'val_acc'])
In [327]:
# 훈련과 검증 정확도 그래프 그리기
plt.clf()
acc = history.history['accuracy']
val_acc = history.history['val_accuracy']
plt.plot(epochs, acc, 'bo', label='Training acc')
plt.plot(epochs, val_acc, 'b', label='Validation acc')
plt.title('Training and Validation accuracy')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
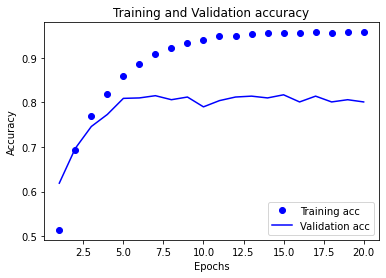
(참고) 배치 사이즈 vs 에포크 vs 반복의 차이¶
- batch size
- 하나의 소 그룹에 속하는 데이터 수
- 전체 훈련 데이터를 작게 나누는 이유는, 훈련 데이터를 통째로 신경망에 넣으면 비효율적 리소스 사용으로 학습시간이 오래걸리기 때문
- batch_size=100이라면, 훈련 데이터 700개가 미니 배치 100개씩 나뉘어진 것
- epoch
- epoch는 전체 훈련 데이터가 신경망을 통과한 횟수
- 1-epoch는 전체 훈련 데이터가 하나의 신경망에 적용되어, 순전파와 역전파를 통해 신경망을 한번 통과했다는 의미
- iteration
- 1-epoch를 마치는데 필요한 미니배치의 개수이다. 즉, 1-epoch를 마치는데 필요한 파라미터 업데이트 횟수이다.
- 각 미니 배치마다 파라미터 업데이터가 한번씩 진행되므로, 예를 들어 700개의 훈련 데이터를 100개씩 7개의 미니배치로 나누었을 때, 1-epoch를 위해서는 7-iteration이 필요하므로, 7번의 파라미터 업데이터가 진행된다.
In [ ]:
# epoch=9로 새로운 모델을 훈련하고, 테스트 세트에서 평가하자
model = models.Sequential()
model.add(layers.Dense(64, activation='relu', input_shape=(10000,)))
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(46, activation='softmax'))
model.compile(optimizer='rmsprop', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(partial_x_train, partial_y_train, epochs=9, batch_size=512,
validation_data=(x_val,y_val))
results = model.evaluate(x_test, one_hot_test_labels)
Epoch 1/9
16/16 [==============================] - 2s 62ms/step - loss: 2.5290 - accuracy: 0.5227 - val_loss: 1.6477 - val_accuracy: 0.6470
Epoch 2/9
16/16 [==============================] - 1s 49ms/step - loss: 1.3605 - accuracy: 0.7126 - val_loss: 1.2851 - val_accuracy: 0.7010
Epoch 3/9
16/16 [==============================] - 1s 48ms/step - loss: 1.0261 - accuracy: 0.7709 - val_loss: 1.1188 - val_accuracy: 0.7510
Epoch 4/9
16/16 [==============================] - 1s 49ms/step - loss: 0.8143 - accuracy: 0.8218 - val_loss: 1.0150 - val_accuracy: 0.7770
Epoch 5/9
16/16 [==============================] - 1s 49ms/step - loss: 0.6461 - accuracy: 0.8622 - val_loss: 0.9461 - val_accuracy: 0.7940
Epoch 6/9
16/16 [==============================] - 1s 51ms/step - loss: 0.5142 - accuracy: 0.8895 - val_loss: 0.9048 - val_accuracy: 0.8150
Epoch 7/9
16/16 [==============================] - 1s 50ms/step - loss: 0.4118 - accuracy: 0.9156 - val_loss: 0.8685 - val_accuracy: 0.8120
Epoch 8/9
16/16 [==============================] - 1s 51ms/step - loss: 0.3359 - accuracy: 0.9285 - val_loss: 0.8688 - val_accuracy: 0.8210
Epoch 9/9
16/16 [==============================] - 1s 50ms/step - loss: 0.2773 - accuracy: 0.9402 - val_loss: 0.8564 - val_accuracy: 0.8250
71/71 [==============================] - 0s 4ms/step - loss: 0.9815 - accuracy: 0.7850
In [330]:
# (참고) 불균형 데이터셋을 무작위로 분류시 18%의 정확도가 나온다.
import copy
test_labels_copy = copy.copy(test_labels)
np.random.shuffle(test_labels_copy)
hits_array = np.array(test_labels_copy)
hits_array = np.array(test_labels) == np.array(test_labels_copy)
float(np.sum(hits_array)) / len(test_labels)
Out[330]:
0.18744434550311664
In [331]:
# 훈련한 모델로 새로운 데이터 예측하기
predictions = model.predict(x_test)
print(predictions[0].shape)
print(np.sum(predictions[0]))
print(np.argmax(predictions[0]))
(46,)
1.0000001
3
In [332]:
# 원-핫 인코딩 말고 정수 텐서로 변환해서 레이블을 인코딩해보자.
y_train = np.array(train_labels)
y_test = np.array(test_labels)
이 방식을 사용하려면 손실함수 하나만 바꾸면 된다. sparse_categorical_crossentropy 사용
In [333]:
model.compile(optimizer='rmsprop', loss='sparse_categorical_crossentropy',metrics=['acc'])
In [ ]:
# 너무 작은 중간층을 사용해서 정보병목이 일어나면 정확도가 얼마나 떨어지는지 확인해보자.
model = models.Sequential()
model.add(layers.Dense(64,activation='relu',input_shape=(10000,)))
model.add(layers.Dense(4,activation='relu'))
model.add(layers.Dense(46,activation='softmax'))
model.compile(optimizer='rmsprop', loss='categorical_crossentropy',metrics=['accuracy'])
model.fit(partial_x_train, partial_y_train, epochs=20, batch_size=128, validation_data=(x_val,y_val)
,verbose=0)
71/71 [==============================] - 0s 3ms/step - loss: 1.9954 - accuracy: 0.6955
회귀 문제 - 주택 가격 예측 예제¶
- 분류 문제는 " 입력 데이터 포인트의 개별적인 레이블 하나를 예측하는 것"이 목적
- 회귀 문제는 개별적인 레이블 대신에 "연속적인 값을 예측"하는 것이 목적
- ex. 기상 데이터로 내일 기온 예측
In [337]:
from keras.datasets import boston_housing
(train_data, train_targets), (test_data, test_targets) = boston_housing.load_data()
print(train_data.shape)
print(test_data.shape)
# 13개의 수치 특성
(404, 13)
(102, 13)
In [338]:
print(train_targets[:10])
# 타깃인 '주택의 중간가격'은 천달러 단위이며, 1만 달러에서 5만 달러 사이 값을 가짐
[15.2 42.3 50. 21.1 17.7 18.5 11.3 15.6 15.6 14.4]
In [339]:
# 데이터 정규화
mean = train_data.mean(axis=0)
train_data -= mean
std = train_data.std(axis=0)
train_data /= std
test_data -= mean
test_data /= std
#주의 - 머신러닝 과정에서는 데이터 정규화와같은 간단한 작업일지라도
# 절대 과정에서 테스트 데이터로 계산해서는 안된다. 다 훈련 데이터로 계산하기
In [340]:
# 모델 정의 (샘플 개수가 적어서 2개의 은닉층을 가진 작은 네트워크를 구성해 사용해보자 )
from keras import models
from keras import layers
def build_model():
model = models.Sequential()
model.add(layers.Dense(64, activation='relu', input_shape = (train_data.shape[1],)))
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(1)) # 여기서는 시그모이드 사용X , '순수 선형층'으로 0과1사이 값이 아닌, 자유로운 학습 가능
model.compile(optimizer='rmsprop', loss='mse', metrics=['mae']) #손실함수는 MSE 사용 / 훈련동안에는 평균절대오차MAE 사용
return model
#MAE가 0.5라면 예측이 500달러정도 차이가 난다는 의미
In [341]:
# 데이터 포인트가 많지 않기 때문에 K-fold cv 사용
import numpy as np
k = 4
num_val_samples = len(train_data) // k
num_epochs = 100
all_scores = [ ]
for i in range(k):
print('처리중인 폴드 #', i)
val_data = train_data[i*num_val_samples: (i+1)*num_val_samples]
val_targets = train_targets[i*num_val_samples: (i+1)*num_val_samples]
partial_train_data = np.concatenate([train_data[:i*num_val_samples],
train_data[(i+1)*num_val_samples:]],axis=0)
partial_train_targets = np.concatenate([train_targets[:i*num_val_samples],
train_targets[(i+1)*num_val_samples:]],axis=0)
처리중인 폴드 # 0
처리중인 폴드 # 1
처리중인 폴드 # 2
처리중인 폴드 # 3
In [349]:
# 케라스 모델 구성 (컴파일 포함)
model = build_model()
model.fit(partial_train_data, partial_train_targets, epochs = 100, batch_size=1, verbose=0)
val_mse, val_mae = model.evaluate(val_data, val_targets, verbose=0)
all_scores.append(val_mae)
# verbose=0설정시 훈련 과정 출력되지 않음
In [350]:
all_scores
Out[350]:
[2.5921385288238525, 2.8203396797180176]
In [347]:
np.mean(all_scores)
Out[347]:
2.5921385288238525
In [352]:
# epochs=500으로 설정하고 얼마나 모델이 개선되는지 확인해보자.
num_epochs = 500
all_mae_histories = [ ]
for i in range(k):
print('처리중인 폴드 #', i)
val_data = train_data[ i * num_val_samples: (i+1)* num_val_samples]
val_targets = train_targets[ i * num_val_samples: (i+1) * num_val_samples]
partial_train_data = np.concatenate( [train_data[:i*num_val_samples:]],axis=0)
partial_train_targets = np.concatenate([train_targets[:i*num_val_samples],
train_targets[(i+1)*num_val_samples:]],axis=0)
처리중인 폴드 # 0
처리중인 폴드 # 1
처리중인 폴드 # 2
처리중인 폴드 # 3
In [353]:
#케라스 모델 구성(컴파일 포함)
model = build_model()
history = model.fit(partial_train_data, partial_train_targets, validation_data=(val_data,val_targets),
epochs = num_epochs, batch_size=1, verbose=0)
mae_history = history.history['val_mae']
all_mae_histories.append(mae_history)
In [268]:
history.history.keys() #key에 val_mae로 되어있는데 val_mean_absoluate_error로 불러와서 에러났었음
Out[268]:
dict_keys(['loss', 'mae', 'val_loss', 'val_mae'])
In [354]:
# k-fold 검증 점수 평균 기록
average_mae_history = [np.mean([x[i] for x in all_mae_histories]) for i in range(num_epochs)]
In [355]:
# 검증점수 그래프
import matplotlib.pyplot as plt
plt.plot(range(1,len(average_mae_history) +1), average_mae_history)
plt.xlabel('Epochs')
plt.ylabel('Valiation MAE')
plt.show()
In [356]:
# 그래프 더 자세히 보기 (다른 부분과 스케일이 심하게 다른 첫 10개의 데이터 제외, 부드러운
# 곡선을 얻기위해 각 포인트를 이전 포인트의 지수 이동 평균으로 대체)
def smooth_curve(points, factor=0.9):
smoothed_points = []
for point in points:
if smoothed_points:
previous = smoothed_points[-1]
smoothed_points.append(previous*factor + point*(1-factor))
else:
smoothed_points.append(point)
return smoothed_points # FOR 문하고 RETURN문하고 같은 위치에 있지않아서 그래프 출력안됐었음
smooth_mae_history = smooth_curve(average_mae_history[10:])
plt.plot(range(1,len(smooth_mae_history)+1), smooth_mae_history)
plt.xlabel('Epochs')
plt.ylabel('Validation MAE')
plt.show()
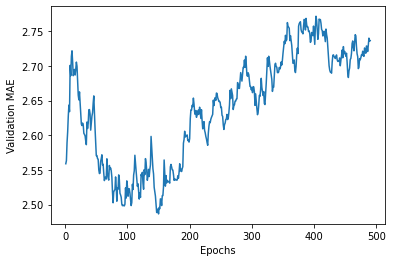
model = build_model() #새롭게 컴파일된 모델 얻기
model.fit(train_data, train_targets,epochs=80, batch_size=16, verbose=0) #전체 데이터로 훈련
test_mse_score, test_mae_score = model.evaluate(test_data, test_targets)
4/4 [==============================] - 0s 4ms/step - loss: 19.2894 - mae: 2.6564
test_mae_score
2.656440258026123
'데이터 분석 > 케라스 창시자에게 배우는 딥러닝' 카테고리의 다른 글
[5] 케라스 창시자에게 배우는 딥러닝 - 5. 컴퓨터 비전을 위한 딥러닝( 합성곱 신경망 소개, MaxPooling, 고양이vs강아지 분류 예제, 데이터 증식) (0) | 2022.08.16 |
---|---|
[4] 케라스 창시자에게 배우는 딥러닝 - 4. (머신러닝) 분류, 특성 공학, 특성 학습, 과/소적합, 해결법(가중치 규제,드롭아웃 추가..) (0) | 2022.08.11 |
[3] 케라스 창시자에게 배우는 딥러닝 - 신경망 시작하기(분류/예측 예제 풀어보기) (0) | 2022.08.08 |
[2] 케라스 창시자에게 배우는 딥러닝 - 신경망의 수학적 구성 요소 (0) | 2022.08.08 |
[1] 케라스 창시자에게 배우는 딥러닝 - 딥러닝의 기초 (이론) (0) | 2022.07.28 |
Comments